Don't always sleep while waiting for exited threads
Rather than poll our exitthread Queue in a non-blocking fashion and always sleep for 1 second inbetween, simply call it in a blocking fashion which will return immediately when a thread has exited. This is somewhat faster as we don't do unnecessary sleeps after a thread exited. Do note that we need to specify some timeout value here (the 60 chosen is pretty arbitary, but what the value exactly is, is not that important, it could be any positive value) in order to make the Queue.get() call work with SIGINT (cf http://bugs.python.org/issue1360). Signed-off-by: Sebastian Spaeth <Sebastian@SSpaeth.de> Signed-off-by: Nicolas Sebrecht <nicolas.s-dev@laposte.net>
This commit is contained in:
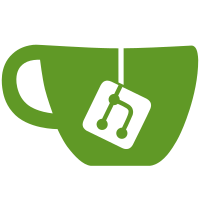
committed by
Nicolas Sebrecht
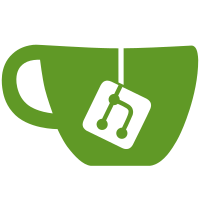
parent
1b36c314c6
commit
0cdfffa04d
@ -101,22 +101,31 @@ def initexitnotify():
|
||||
pass
|
||||
|
||||
def exitnotifymonitorloop(callback):
|
||||
"""Enter an infinite "monitoring" loop. The argument, callback,
|
||||
defines the function to call when an ExitNotifyThread has terminated.
|
||||
That function is called with a single argument -- the ExitNotifyThread
|
||||
that has terminated. The monitor will not continue to monitor for
|
||||
other threads until the function returns, so if it intends to perform
|
||||
long calculations, it should start a new thread itself -- but NOT
|
||||
an ExitNotifyThread, or else an infinite loop may result. Furthermore,
|
||||
the monitor will hold the lock all the while the other thread is waiting.
|
||||
"""An infinite "monitoring" loop watching for finished ExitNotifyThread's.
|
||||
|
||||
:param callback: the function to call when a thread terminated. That
|
||||
function is called with a single argument -- the
|
||||
ExitNotifyThread that has terminated. The monitor will
|
||||
not continue to monitor for other threads until
|
||||
'callback' returns, so if it intends to perform long
|
||||
calculations, it should start a new thread itself -- but
|
||||
NOT an ExitNotifyThread, or else an infinite loop
|
||||
may result.
|
||||
Furthermore, the monitor will hold the lock all the
|
||||
while the other thread is waiting.
|
||||
:type callback: a callable function
|
||||
"""
|
||||
global exitthreads
|
||||
while 1: # Loop forever.
|
||||
while 1:
|
||||
# Loop forever and call 'callback' for each thread that exited
|
||||
try:
|
||||
thrd = exitthreads.get(False)
|
||||
# we need a timeout in the get() call, so that ctrl-c can throw
|
||||
# a SIGINT (http://bugs.python.org/issue1360). A timeout with empty
|
||||
# Queue will raise `Empty`.
|
||||
thrd = exitthreads.get(True, 60)
|
||||
callback(thrd)
|
||||
except Empty:
|
||||
time.sleep(1)
|
||||
pass
|
||||
|
||||
def threadexited(thread):
|
||||
"""Called when a thread exits."""
|
||||
|
Reference in New Issue
Block a user