mirror of
https://github.com/rad4day/Waybar.git
synced 2023-12-21 10:22:59 +01:00
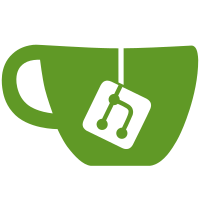
Mouse-over tooltips set on the label only appear once the mouse hovers over exactly the label. Other apps (e.g. firefox) show the tooltip once the pointer hovers the button. Not solely its label. With this commit we get the same behaviour.
56 lines
1.3 KiB
C++
56 lines
1.3 KiB
C++
#include "modules/sway/mode.hpp"
|
|
|
|
#include <spdlog/spdlog.h>
|
|
|
|
namespace waybar::modules::sway {
|
|
|
|
Mode::Mode(const std::string& id, const Json::Value& config)
|
|
: AButton(config, "mode", id, "{}", 0, true) {
|
|
ipc_.subscribe(R"(["mode"])");
|
|
ipc_.signal_event.connect(sigc::mem_fun(*this, &Mode::onEvent));
|
|
// Launch worker
|
|
ipc_.setWorker([this] {
|
|
try {
|
|
ipc_.handleEvent();
|
|
} catch (const std::exception& e) {
|
|
spdlog::error("Mode: {}", e.what());
|
|
}
|
|
});
|
|
dp.emit();
|
|
}
|
|
|
|
void Mode::onEvent(const struct Ipc::ipc_response& res) {
|
|
try {
|
|
std::lock_guard<std::mutex> lock(mutex_);
|
|
auto payload = parser_.parse(res.payload);
|
|
if (payload["change"] != "default") {
|
|
if (payload["pango_markup"].asBool()) {
|
|
mode_ = payload["change"].asString();
|
|
} else {
|
|
mode_ = Glib::Markup::escape_text(payload["change"].asString());
|
|
}
|
|
} else {
|
|
mode_.clear();
|
|
}
|
|
dp.emit();
|
|
} catch (const std::exception& e) {
|
|
spdlog::error("Mode: {}", e.what());
|
|
}
|
|
}
|
|
|
|
auto Mode::update() -> void {
|
|
if (mode_.empty()) {
|
|
event_box_.hide();
|
|
} else {
|
|
label_->set_markup(fmt::format(format_, mode_));
|
|
if (tooltipEnabled()) {
|
|
button_.set_tooltip_text(mode_);
|
|
}
|
|
event_box_.show();
|
|
}
|
|
// Call parent update
|
|
AButton::update();
|
|
}
|
|
|
|
} // namespace waybar::modules::sway
|