mirror of
https://github.com/rad4day/Waybar.git
synced 2025-07-13 06:32:30 +02:00
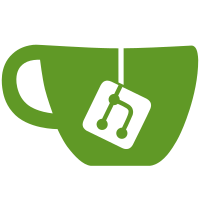
Last part of the rework of handleEvents(), this time we take the getExternalInterface() function and add it to the handleEvents() function. That way, waybar can react immediately when a new "external interface" is available and doesn't need to probe. Also that avoid to have two different functions consuming from the same socket and we don't need to recode some of the functions that are already available via libnl (to send and receive messages).
83 lines
2.2 KiB
C++
83 lines
2.2 KiB
C++
#pragma once
|
|
|
|
#include <arpa/inet.h>
|
|
#include <fmt/format.h>
|
|
#include <linux/nl80211.h>
|
|
#include <netlink/genl/ctrl.h>
|
|
#include <netlink/genl/genl.h>
|
|
#include <netlink/netlink.h>
|
|
#include <sys/epoll.h>
|
|
#include "ALabel.hpp"
|
|
#include "util/sleeper_thread.hpp"
|
|
#ifdef WANT_RFKILL
|
|
#include "util/rfkill.hpp"
|
|
#endif
|
|
|
|
namespace waybar::modules {
|
|
|
|
class Network : public ALabel {
|
|
public:
|
|
Network(const std::string&, const Json::Value&);
|
|
~Network();
|
|
auto update() -> void;
|
|
|
|
private:
|
|
static const uint8_t MAX_RETRY = 5;
|
|
static const uint8_t EPOLL_MAX = 200;
|
|
|
|
static int handleEvents(struct nl_msg*, void*);
|
|
static int handleEventsDone(struct nl_msg*, void*);
|
|
static int handleScan(struct nl_msg*, void*);
|
|
|
|
void askForStateDump(void);
|
|
|
|
void worker();
|
|
void createInfoSocket();
|
|
void createEventSocket();
|
|
void parseEssid(struct nlattr**);
|
|
void parseSignal(struct nlattr**);
|
|
void parseFreq(struct nlattr**);
|
|
bool associatedOrJoined(struct nlattr**);
|
|
bool checkInterface(std::string name);
|
|
auto getInfo() -> void;
|
|
const std::string getNetworkState() const;
|
|
void clearIface();
|
|
bool wildcardMatch(const std::string& pattern, const std::string& text) const;
|
|
|
|
int ifid_;
|
|
sa_family_t family_;
|
|
struct sockaddr_nl nladdr_ = {0};
|
|
struct nl_sock* sock_ = nullptr;
|
|
struct nl_sock* ev_sock_ = nullptr;
|
|
int efd_;
|
|
int ev_fd_;
|
|
int nl80211_id_;
|
|
std::mutex mutex_;
|
|
|
|
bool want_route_dump_;
|
|
bool want_link_dump_;
|
|
bool want_addr_dump_;
|
|
bool dump_in_progress_;
|
|
|
|
unsigned long long bandwidth_down_total_;
|
|
unsigned long long bandwidth_up_total_;
|
|
|
|
std::string state_;
|
|
std::string essid_;
|
|
std::string ifname_;
|
|
std::string ipaddr_;
|
|
std::string netmask_;
|
|
int cidr_;
|
|
int32_t signal_strength_dbm_;
|
|
uint8_t signal_strength_;
|
|
uint32_t frequency_;
|
|
|
|
util::SleeperThread thread_;
|
|
util::SleeperThread thread_timer_;
|
|
#ifdef WANT_RFKILL
|
|
util::Rfkill rfkill_;
|
|
#endif
|
|
};
|
|
|
|
} // namespace waybar::modules
|