mirror of
https://github.com/rad4day/Waybar.git
synced 2023-12-21 10:22:59 +01:00
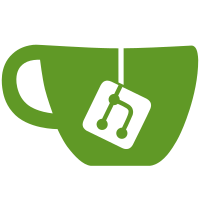
Subscribe for mouse scroll events on the pulseaudio widget and change volume when event is received. Scroll up increments the volume and scroll down decrements it. These events are only subscibed when there are no user defined commands present for them. Signed-off-by: Harish Krupo <harishkrupo@gmail.com>
40 lines
1.0 KiB
C++
40 lines
1.0 KiB
C++
#pragma once
|
|
|
|
#include <fmt/format.h>
|
|
#include <pulse/pulseaudio.h>
|
|
#include <pulse/volume.h>
|
|
#include <algorithm>
|
|
#include "ALabel.hpp"
|
|
|
|
namespace waybar::modules {
|
|
|
|
class Pulseaudio : public ALabel {
|
|
public:
|
|
Pulseaudio(const Json::Value&);
|
|
~Pulseaudio();
|
|
auto update() -> void;
|
|
private:
|
|
static void subscribeCb(pa_context*, pa_subscription_event_type_t,
|
|
uint32_t, void*);
|
|
static void contextStateCb(pa_context*, void*);
|
|
static void sinkInfoCb(pa_context*, const pa_sink_info*, int, void*);
|
|
static void serverInfoCb(pa_context*, const pa_server_info*, void*);
|
|
static void volumeModifyCb(pa_context*, int, void*);
|
|
bool handleScroll(GdkEventScroll* e);
|
|
|
|
const std::string getPortIcon() const;
|
|
|
|
pa_threaded_mainloop* mainloop_;
|
|
pa_mainloop_api* mainloop_api_;
|
|
pa_context* context_;
|
|
uint32_t sink_idx_{0};
|
|
uint16_t volume_;
|
|
pa_cvolume pa_volume_;
|
|
bool muted_;
|
|
std::string port_name_;
|
|
std::string desc_;
|
|
bool scrolling_;
|
|
};
|
|
|
|
} // namespace waybar::modules
|