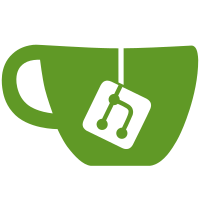
Previously we would output: Folder sync sspaeth.de[INBOX.INBOX201004]: Syncing INBOX.INBOX201004: IMAP -> Maildir Folder sync sspaeth.de[INBOX.INBOX201006]: Syncing INBOX.INBOX201006: IMAP -> Maildir Folder sync sspaeth.de[INBOX.INBOX201009]: Syncing INBOX.INBOX201009: IMAP -> Maildir which is very repetitive and cluttered. By naming the folder sync threads just according to the account and not the folder, the output looks much nicer: Folder sync [sspaeth.de]: Syncing INBOX.INBOX201004: IMAP -> Maildir Syncing INBOX.INBOX201006: IMAP -> Maildir Syncing INBOX.INBOX201009: IMAP -> Maildir If syncing multiple accounts in parallel, we will still get headers indicating the account: Folder sync [sspaeth.de]: Syncing INBOX: IMAP -> Maildir Syncing INBOX.INBOX201006: IMAP -> Maildir Folder sync [gmail]: Syncing INBOX: IMAP -> Maildir This is a small fix that makes the output much nicer in my opinion. Also don't output the thread name if we are in the MainThread, e.g. when we output the initial offlineimap banner. Signed-off-by: Sebastian Spaeth <Sebastian@SSpaeth.de> Signed-off-by: Nicolas Sebrecht <nicolas.s-dev@laposte.net>
72 lines
2.5 KiB
Python
72 lines
2.5 KiB
Python
# TTY UI
|
|
# Copyright (C) 2002 John Goerzen
|
|
# <jgoerzen@complete.org>
|
|
#
|
|
# This program is free software; you can redistribute it and/or modify
|
|
# it under the terms of the GNU General Public License as published by
|
|
# the Free Software Foundation; either version 2 of the License, or
|
|
# (at your option) any later version.
|
|
#
|
|
# This program is distributed in the hope that it will be useful,
|
|
# but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
# GNU General Public License for more details.
|
|
#
|
|
# You should have received a copy of the GNU General Public License
|
|
# along with this program; if not, write to the Free Software
|
|
# Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA 02110-1301 USA
|
|
|
|
from UIBase import UIBase
|
|
from getpass import getpass
|
|
import select, sys
|
|
from threading import *
|
|
|
|
class TTYUI(UIBase):
|
|
def __init__(s, config, verbose = 0):
|
|
UIBase.__init__(s, config, verbose)
|
|
s.iswaiting = 0
|
|
s.outputlock = Lock()
|
|
s._lastThreaddisplay = None
|
|
|
|
def isusable(s):
|
|
return sys.stdout.isatty() and sys.stdin.isatty()
|
|
|
|
def _display(s, msg):
|
|
s.outputlock.acquire()
|
|
try:
|
|
#if the next output comes from a different thread than our last one
|
|
#add the info.
|
|
#Most look like 'account sync foo' or 'Folder sync foo'.
|
|
try:
|
|
threadname = currentThread().name
|
|
except AttributeError:
|
|
threadname = currentThread().getName()
|
|
if (threadname == s._lastThreaddisplay \
|
|
or threadname == 'MainThread'):
|
|
print " %s" % msg
|
|
else:
|
|
print "%s:\n %s" % (threadname, msg)
|
|
s._lastThreaddisplay = threadname
|
|
|
|
sys.stdout.flush()
|
|
finally:
|
|
s.outputlock.release()
|
|
|
|
def getpass(s, accountname, config, errmsg = None):
|
|
if errmsg:
|
|
s._msg("%s: %s" % (accountname, errmsg))
|
|
s.outputlock.acquire()
|
|
try:
|
|
return getpass("%s: Enter password: " % accountname)
|
|
finally:
|
|
s.outputlock.release()
|
|
|
|
def mainException(s):
|
|
if isinstance(sys.exc_info()[1], KeyboardInterrupt) and \
|
|
s.iswaiting:
|
|
sys.stdout.write("Timer interrupted at user request; program terminating. \n")
|
|
s.terminate()
|
|
else:
|
|
UIBase.mainException(s)
|
|
|